This is the first in a series of walkthroughs for beginners in .NET that show how to integrate Adobe Flex applications with .NET servers. This first walkthrough guides you through the tasks involved in creating a simple Flex application that shows the growth rates for the US economy in a simple line chart whose data values are retrieved from a .NET servlet. Later walkthroughs will show you how to make your .NET servlets respond to events generated by Flex applicaitons and how to serialize complex server-side .NET data types so that they can be consumed by Flex.
To complete these walkthroughs you will need:
- Adobe Flex Builder V2 Beta3 (trial) or Flex SDK V2 Beta3 (free)
- Adobe Flash Player V9 Beta3 (free)
- Microsoft Visual Web Developer 2005 Express Edition or the .NET V2.0 SDK (both free)
- Microsoft Windows
The finished Flex application looks like this.

Source: IMF
The application works like this. When the browser requests the Flex application's HTML page, the HTML page and the compiled Flex SWF embedded in the HTML page are download from the webserver. The browser then runs the Flex SWF in the Flash Player plug-in. The Flex SWF makes an HTTP request to the .NET servlet on the same webserver to obtain the data for the chart. The .NET servlet generates an HTTP response with the data formatted in XML. When the response is received by the SWF the chart line is drawn.
This is how you build this simple Flex charting application.
Step 1: Create the .NET Servlet
The first step in building the application is to create the servlet that will respond to the Flex application's request for the growth rate data. ASP.NET is perfect for generating responses to HTTP requests but unfortunately is designed to generate HTML, not XML. Nonetheless, with a little persuasion, ASP.NET can be made to generate pure XML responses programatically. Microsoft hasn't invented a name yet for an ASP.NET page that generates dynamic XML but in Java a page like this would probably be called a servlet so I use that term here.
There's no special project type in Visual Web Developer for an XML servlet so we'll use a regular ASP.NET Web Site project and modify the web application so that it responds with XML instead of HTML. Create a new web site as shown here:
Select File system if you're using Visual Web Developer's build-in web server and ...\GrowthServlet as the web site's location in the file system. Select the language. I've used Visual C# in this example but you can use whichever of the .NET languages you are most familiar with.
Step 2: Remove the HTML from the Servlet's ASPX Page
By default Visual Web Developer includes some HTML in any new web page. Remove all of the HTML from the servlet's Default.aspx page . Leave the page directive in place (the element enclosed by the <%@ Page %>tags) but strip out all the other elements. Add ContentType="text/xml" to the page directive.
The finished Default.aspx page should look like this.<%@ Page Language="c#" ContentType="text/xml"
CodeFile="Default.aspx.cs" Inherits="_Default" %>
Step 3: Modify the Servlet's Code Behind to generate the XML
The next step is to add code to the Page_Load event handler in the servlet's code behind file to generate the XML programatically. The simplest way to generate XML programatically is to use ASP.NET's Response.Write() to write XML literals to the HTTP response stream. The following example shows this approach:using System;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
Response.Write( "<GrowthRates>" ) ;
Response.Write( "<Rate>36.5</Rate>" ) ;
Response.Write( "<Rate>36.1</Rate>" ) ;
Response.Write( "<Rate>36.4</Rate>" ) ;
Response.Write( "<Rate>36.7</Rate>" ) ;
Response.Write( "<Rate>37.1</Rate>" ) ;
Response.Write( "<Rate>37.4</Rate>" ) ;
Response.Write( "<Rate>36.6</Rate>" ) ;
Response.Write( "</GrowthRates>" ) ;
}
}
Step 4: Test the Servlet in a Browser
Check the ASP.NET servlet generates the XML you're expecting by hitting F5 in Visual Web Developer. The first time you do this Visual Web Developer will show the following dialogue to ask if you want to create a web.config file to enable the debugger.
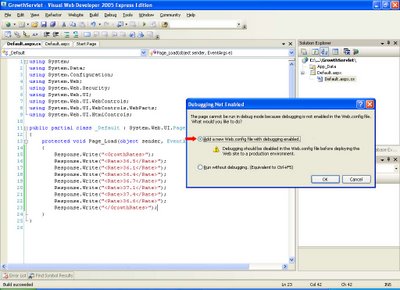
Select Add a new web.config file and click OK. This will launch your default browser and show the XML generated by the servlet.

If your XML looks like the figure then you're ready to build the Flex application. Take a note of the port number that the ASP.NET Development Server is using for the servlet as you'll need that in your Flex application.
Step 5: Create the Flex Application
Create the Flex application GrowthRates.mxml using either Flex Builder or Notepad. The HTTPService component retrieves the data from the servlet and the LineChart component uses the results of the HTTPService as the data series for the chart:
<?xml version="1.0" encoding="utf-8"?>
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml"
backgroundColor="white" backgroundImage=""
creationComplete="this.servlet.send();">
<mx:HTTPService id="servlet"
url="http://localhost:1317/GrowthServlet/Default.aspx" />
<mx:LineChart showDataTips="true"
dataProvider="{this.servlet.lastResult.GrowthRates.Rate}">
<mx:series>
<mx:LineSeries />
</mx:series>
<mx:verticalAxis>
<mx:LinearAxis baseAtZero="false" />
</mx:verticalAxis>
</mx:LineChart>
</mx:Application>
The finished Flex application should look like this.

The data tips should pop up as you mouse over the data points on the chart. It realy is as easy as that.
15 comments:
Gene,
Do you know of a way to make the xml page more dynamic by using a sql query??
Thanks
Graeme I am sorry, I am not sure why I called you Gene, typing too fast.
Yes, although you'll have to wait until I get back from vacation for the next episodes. Stay tuned.
PS I quite like Gene.
Have you seen Part 2?
Part 2 doesn't cover databases but does take it one stage further.
My plan is to cover responding to input from Flex next, then cover the serialization of .NET collections and then cover databases and DataSets.
(Since you like Gene)
Gene,
Nice Tutorial - great help and direction for a quick start. I'm gonna see if I can figure out how to do this from a SQL query.
Thanks again.
mike
why i come out error messages?
it's like validation error message. how come i do?
It's likely that this example doesn't work any more. I wrote it more than two years ago for the beta of Flex 2.
Works For Me Graeme. VS2003 is a little fussy when you take out the HTML Tags and you must generate a new page rather than chaging wthe web form name but all else OK. Flex does not seem to care wether there is an XML header or not.
ie <?xml version="1.0" encoding="utf-8 ?>
However displaying the xml in IE seems to.
Thanx Graeme for this wonderful article, I have used httpHandler in asp.net instead of default.aspx page and have fetched the data from database into DataSet and then converted the DataSet to xml String. Only xmlstring generation was what i didnt liked but its all about .net part, above all your article has helped me a lot,. With lots of thanx and god bless you.
regards
Daman
Graeme,
You should be using a HTTP Handler to do what you call a .NET servlet. Its the closest equivalent of the servlet in the .NET world.
Thanks Greame,
It was an nice tutorial on using flex with Dot net side...I just want to know the difference between accessing dot net page using httpservice or by webservice??
Vipin,
It simply depends what you're trying to do. A simple .NET "servlet" can return anything (it doesn't have to be XML, it could be a binary response for example) whereas a .NET WebService obviously has to return a valid SOAP response. In that sense a simple servlet is more "flexible" but it also more low-level, which isn't always what your want. Horses for coarses.
Graeme
Very Clear Cut Explanation....I got so much of confidence,like i can still stand in my team,after reading this post.Thanks a lot for this post.
very nice tutorial.....
Graeme,
Could you give more idea on displaing data set value in the gridview.
Nice work, i got help from this a lot.
Post a Comment