The finished Flex application, which shows the growth rates for the three economic areas, looks like this.
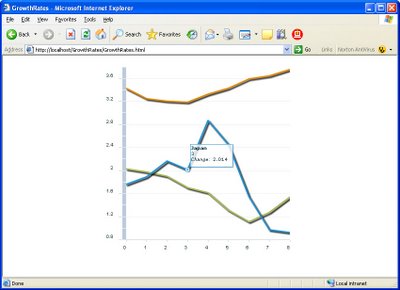
Step 1: Create a new Servlet in Visual Studio
The first step is to create a new servlet to provide the HTTP response to the Flex application's HTTP request. The servlet will be an new web form in the same ASP.NET project you created in Part 1. To add a new form, right-click the project name in Solution Explorer and selecting Add New Item..., Web Form. Name the new form Rates.aspx.
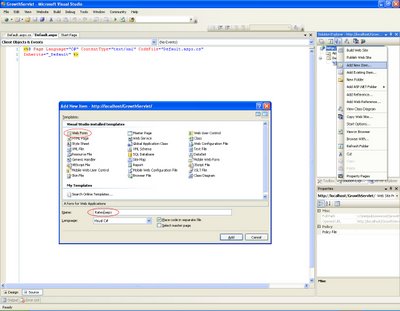
Step 2: Modify the Servlet's ASPX Page to remove the HTML
As we did in Part 1, the next step is to remove all the HTML elements from the servlet's ASPX page. When you create a new web form using Visual Studio it inserts template HTML that we don't need for an XML servlet. Leave the page directive element in place (the line enclosed by the <%@ Page %> tags) but strip out everything else. Add ContentType="text/xml" to the page directive. The finished Rates.aspx page should look like this.
<%@ Page Language="c#" ContentType="text/xml"
CodeFile="Rates.aspx.cs" Inherits="Rates" %>
Step 3: Modify the Servlet's Code Behind to generate the XML
The next step is to add code to the servlet's Page_Load event handler in the servlet's code behind file to generate the required XML programatically. Again we'll just use ASP.NET's Response.Write() to write XML literals directly to the HTTP response stream. Add the following code in bold to Rates.aspx.cs
using System;
public partial class Rates : System.Web.UI.Page
{
private void Page_Load(object sender, EventArgs e)
{
Response.Write( "<GrowthRates>" ) ;
Response.Write( "<Rate US='3.429' Euro='2.030' Japan='1.761'/>" );
Response.Write( "<Rate US='3.248' Euro='1.975' Japan='1.891'/>" );
Response.Write( "<Rate US='3.206' Euro='1.901' Japan='2.166'/>" );
Response.Write( "<Rate US='3.186' Euro='1.703' Japan='2.014'/>" );
Response.Write( "<Rate US='3.325' Euro='1.616' Japan='2.870'/>" );
Response.Write( "<Rate US='3.431' Euro='1.310' Japan='2.451'/>" );
Response.Write( "<Rate US='3.592' Euro='1.110' Japan='1.546'/>" );
Response.Write( "<Rate US='3.645' Euro='1.274' Japan='0.972'/>" );
Response.Write( "<Rate US='3.756' Euro='1.539' Japan='0.924'/>" );
Response.Write( "</GrowthRates>" ) ;
}
}
Step 4: Test the Servlet with IE
You can test that the servlet generates the XML you're expecting by hitting F5 in Visual Studio. This will launch IE. You should see the following screen.
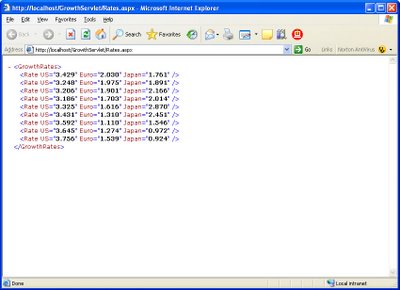
Step 5: Create the Flex Application with Flex Builder
Modify the Flex application from Part1. Change the HTTPService to use the new ASP.NET servlet http://localhost/GrowthServlet/Rates.aspx which will provide the datafor the chart. The Flex application's MXML file should look like this.
<?xml version="1.0" encoding="utf-8"?>
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml"
xmlns="*" backgroundColor="white"
creationComplete="this.servlet.send();">
<mx:HTTPService id="servlet"
url="http://localhost/GrowthServlet/Rates.aspx"/>
<mx:LineChart showDataTips="true" dataProvider="{servlet.result.GrowthRates.Rate}" >
<mx:series>
<mx:LineSeries yField="US" displayName="US" />
<mx:LineSeries yField="Euro" displayName="Euro" />
<mx:LineSeries yField="Japan" displayName="Japan" />
</mx:series>
<mx:verticalAxis>
<mx:LinearAxis baseAtZero="false" name="Change"/>
</mx:verticalAxis>
</mx:LineChart>
</mx:Application>
The finished application should look like the following figure with lines for the US, Euro zone and Japanese growth rates.
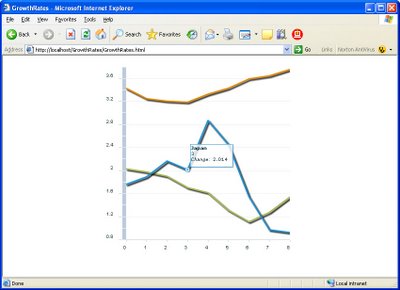
And that's how easy it is.
11 comments:
Informative blog. Thanks.
Regards,
Stock Trader
trading information
Cool blog. But what if I want to use the data entered from flex to perform a query ?
Hi,
I am absolutely amazed at the dynamism of flex on a once a static web of html pages.....
However how do I pull data from an SQL server database and plot in real-time...? Can this be done with FLEX and .NET??
Any ideas or links will be highly appreciated.
Thanks Nd Regards
Tanmay.
There should be no problem hooking this up to a database. Just create your connection, and re-form the resulting data into XML tagged data.
I personally started down the track of making my MSSQL data available through a .net webservice. But returning datasets of data is not good for Flex. So I copied the dataset into an arralist and serialised it into XML. Flex seemed happy with it then.
Pete.
Cool Blog. Can I see one where you send data back to an asp.net servlet?
Hi Flex throwing me the error
Cannot resolve attribute 'name' for component type mx.charts.LinearAxis.
very informative...
I managed to do and application with the help of ur article. I have one doubt, can i assign the information retrieve through http service to any variable??If so, how??
In the abouve article you are assigning the values directly to chart provider right, can we assign it to an Array or ArrayCollection? I tried but couldnt??Is there any other way?
well written blog, and easy to follow. But I have a different problem here in my application. I have developed a flex application which is embedded in an ASP.NET page and needs to get dynamic application parameters from my ASP .NET web page to retrieve server-side data. How should I pass parameters from ASP.NET to flex?
The classic way to do this is to generate the HTML for the "flashvars" attribute of the "object" and "embed" tags programatically in your ASP.NET Page and then access the parameters as dynamic properties of Flex's Application.application object. (Do a google search for flashvars as this is a well-established technique for Flash applications).
Then look for flashvars in the Flex doc set for an explanation of how to access these parameters from Flex.
Alternatively you can get your Flex application to make a separate HTTP request to a separate ASP.NET servlet to get server-side parameters.
Hi,
I just want to say, that the way you explain and describe good. I have yet to see anyone so descriptive like you.
It would be nice to see an example with SQL Server integration.
Thank you very much,
Alks
Hi,
Thanks for your valuable article. I want to know the IIS configuration and the client side configuration of Flex.
So that will deploying also we may not face any problem.
Thank u,
gsaikrishna23@yahoo.co.in
Post a Comment