From a developer's point of view, the run-time environment is similar to Windows.Forms where the dynamic aspects of the UI are written as event handlers in code. Hence developers with experience of developing Windows.Forms applications will be able to leverage their experience. The development environment on the other hand has more in common with ASP.NET, where the static layout of the UI is described in an XML-based markup language. In Flex the programming language is ActionScript, which now a fully-fledged object-oriented programming language like Java and C#.
Best of all, since Flex applicaitons run in the Flash player, animation, drawing, sound and video effects, previously only available to Flash programmers, are now available via the Flex class library to enterprise application developers.
Flex v1.5 required a Java-based Flex server to compile and serve Flex pages on-demand. Flex v2 has a different licensing model which means Flex applications can be pre-compiled and deployed on a regular webserver such as IIS and can be easily integrated with a .NET-based servers tier.
At runtime, Flex applications communicate with the server tier using either XML Web services (SOAP over HTML), REST (XML over HTTP) or Adobe's own proprietary binary protocol (AMF). AMF support on the server is provided by the optional Flex server component called Flex Data Services (previously Flex Enterprise Services). FDS provides support for pub-sub messaging, data push and remote access to Java objects but is currently only available for Java servers. The good news is that for many RIA applications, REST and/or Web services are more than sufficient and since .NET provides good server-side support for XML, SOAP and REST, you can build an server tier to support Flex applicaitons in .NET. If however you need the extra services provided by FDS, you're stuck with Java on the server.
This post is the first in a series that what is possible with Flex and .NET servers. This first walkthrough guides you through the tasks involved in creating a simple Flex application that uses a .NET servlet to provide its data.
To complete this walkthrough you will need:
- Visual Studio (the example screens are VS2005 but no v2.0 features are used)
- An IIS webserver (with ASP.NET enabled)
- Flex Builder 2 or Flex2 SDK (the example is tested with Beta 2)
- Flash Player Plug-in V8.5 (the example is tested with Beta 2)
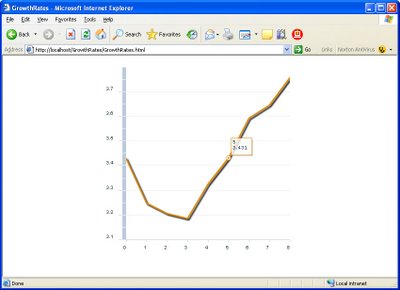
Source: IMF
The pre-compiled Flex application downloads from the webserver and runs in the browser's Flash Player. To render the chart the application makes an HTTP request to the web server for the chart's data. In response, the server generates an HTTP response with the chart's data formatted in XML.
Step 1: Create a .NET Servlet in Visual Studio
The first step in building the application is to create the servlet that will respond to the Flex application's request for the growth rate data. ASP.NET is perfect for generating responses to HTTP requests but unfortunately is designed to generate HTML, not XML. Nonetheless, with a little persuasion, ASP.NET can be made to generate REST responses programatically. Microsoft hasn't invented a name yet for an ASP.NET page that generates dynamic XML but in Java, a page like this would probably be called a servlet so I use that term here.
There's no special project type in Visual Studio for an XML servlet so we'll use a regular ASP.NET Web Site project and modify the web application so that it responds with XML instead of HTML. Create a new web site as shown here:
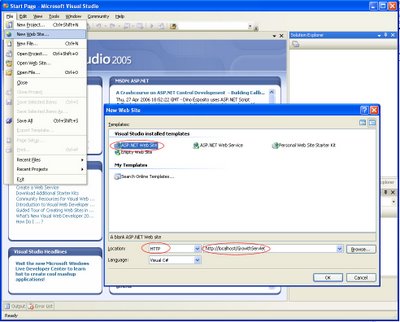
I've used C# in this example but you can use whichever of the .NET languages you are most familiar with. Set the location type as HTTP and path as http://localhost/GrowthServlet. You can put the servlet on any server but note that, for security reasons, Flex, unless you configure it otherwise, will only retrieve data from the same server that it downloaded the Flex application from, so put the servlet on the same server that you plan to deploy the compiled Flex application.
Step 2: Modify the Servlet's ASPX Page to remove the HTML
The next step is to remove all the static HTML from the Default.aspx page that Visual Studio includes by default. Leave the page directive in place (the element enclosed by the <%@ Page %> tags) but strip out all the other elements. Add ContentType="text/xml" to the page directive. The finished Default.aspx page should look like this.
<%@ Page Language="c#" ContentType="text/xml"
CodeFile="Default.aspx.cs" Inherits="_Default" %>
Step 3: Modify the Servlet's Code Behind to generate the XML
The next step is to add code to the Page_Load event handler in the code behind file to generate the XML programatically. The simplest way to generate XML programatically is to use ASP.NET's Response.Write() to write XML literals to the HTTP response stream. The following example shows this approach:
using System;
public partial class _Default : System.Web.UI.Page
{
private void Page_Load(object sender, EventArgs e)
{
Response.Write( "<GrowthRates>" ) ;
Response.Write( "<Rate>36.5</Rate>" ) ;
Response.Write( "<Rate>36.1</Rate>" ) ;
Response.Write( "<Rate>36.4</Rate>" ) ;
Response.Write( "<Rate>36.7</Rate>" ) ;
Response.Write( "<Rate>37.1</Rate>" ) ;
Response.Write( "<Rate>37.4</Rate>" ) ;
Response.Write( "<Rate>36.6</Rate>" ) ;
Response.Write( "</GrowthRates>" ) ;
}
}
Step 4: Test the Servlet in a Browser
Check the ASP.NET servlet generates the XML you're expecting by hitting F5 in Visual Studio. This should launch IE and show the XML generated by the servlet.
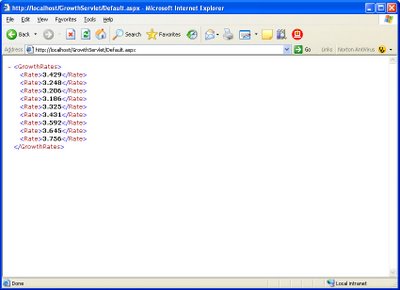
If your XML looks like the figure then you're ready to build the Flex application.
Step 5: Create the Flex Application
Create a Flex application GrowthRates.mxml as shown here using either Flex Bulder or Notepad. The HTTPService component retrieves the data from the servlet and the LineChart component uses the results of the HTTPService as the data series for the chart:
<?xml version="1.0" encoding="utf-8"?>
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml"
xmlns="*" backgroundColor="white"
creationComplete="this.servlet.send();">
<mx:HTTPService id="servlet"
url="http://localhost/GrowthServlet/Default.aspx" />
<mx:LineChart showDataTips="true" dataProvider=
"{this.servlet.result.GrowthRates.Rate}">
<mx:series>
<mx:LineSeries />
</mx:series>
<mx:verticalAxis>
<mx:LinearAxis baseAtZero="false" />
</mx:verticalAxis>
</mx:LineChart>
</mx:Application>
The finished Flex application should look like this.
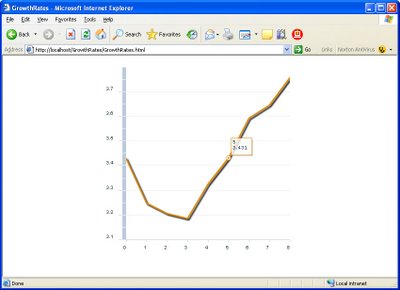
The data tips should pop up as you mouse over the data points on the chart. It realy is as easy as that.
10 comments:
was of great help..thanks a lot!!
Thank you. This was of great help. I cannot believe how little information exists on FLEX 2 and .NET. If anyone is interested, I found the tutorial at
http://flex2-for-dummies.blogspot.com/
to be of use.
Keep up the good work and keep publishing the tutorials!
can you send me the barcode graph sameple also.
thanks in advacne
How can I move the URL ("http://localhost/GrowthServlet/Default.aspx" ) out of the mxml file
and use a named service. I don't want to hard code the url in the swf file. I want to be able
to change the url as I move from local, dev, QA, Production boxes. I am using flex on the
front-end compiled into a swf, and deployed on IIS. I was going to write C# web services for
backend, but I like the .net servlet idea.
There are no "named services" but you can use a relative url rather than an absolute url. For example, you could say url="Default.aspx" and the application will expect to find Default.aspx in the same folder as the swf file.
If you like the idea of .NET "servlets" you should check out the new REST support in WCF in .NET 3.5 that goes by the name of "ADO.NET Data Services"
Can I pass a variable to aspx from flex? I'm trying to generate a query based on the passing variable.
Thanks alot....:)
If your HTTPService's doing a GET or if it's doing a POST with contentType set to "application/x-www-form-urlencoded" then you can retrieve the parameters by simply indexing ASP.NET's Request object. e.g. Request["contactName"].
If you're sending XML then clearly you'll need to parse the XML in the servlet.
Where did you create GrowthRates.html file in the above scenario? In the browser's address bar it shows http://localhost/GrowthServlet/GrowthRates.html.
But you didn't create this file in the above scenario.
it's good to see this information in your post, i was looking the same but there was not any proper resource, thanx now i have the link which i was looking for my research.
Post a Comment